This class is an abstract class. It supplies the interface for objective functions. More...
#include <function.h>
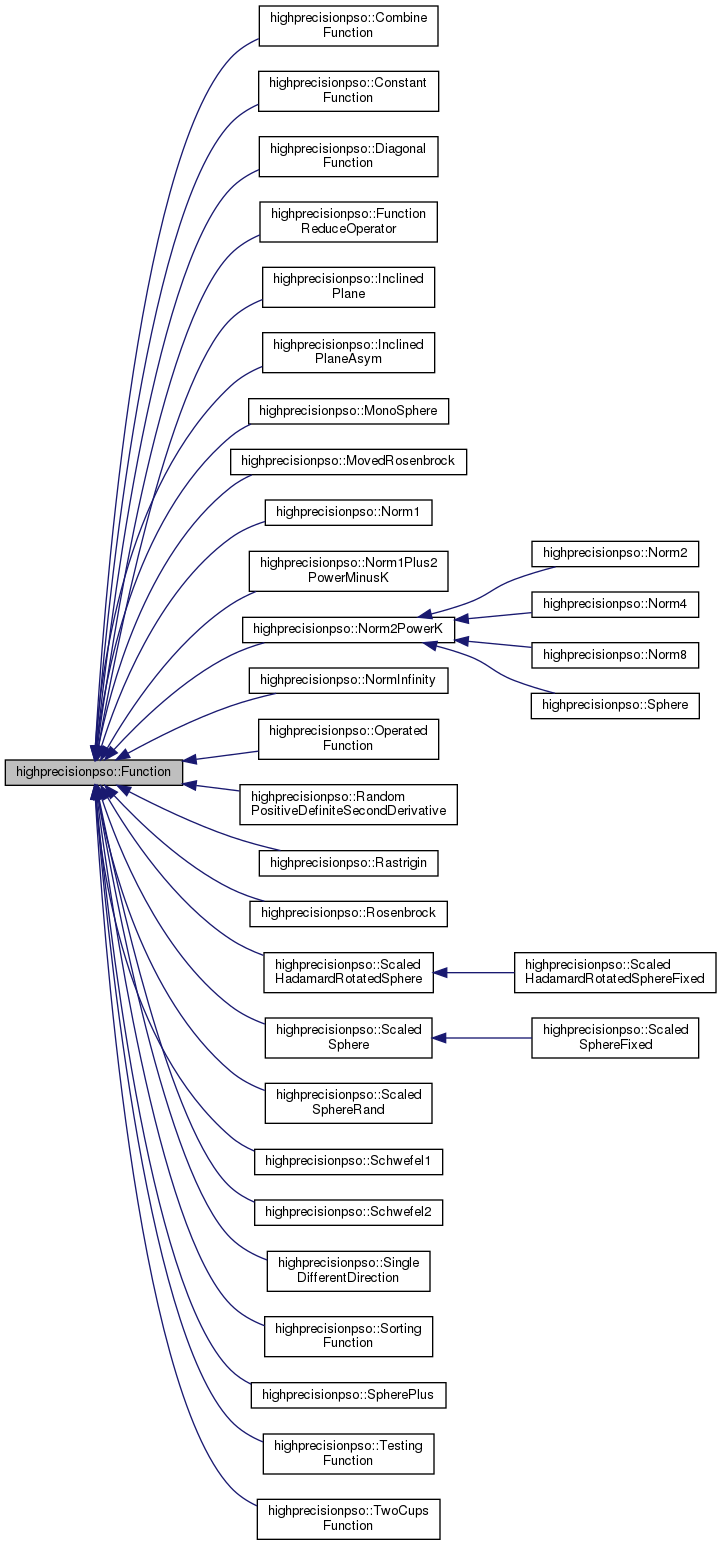
Public Member Functions | |
Function () | |
A constructor. More... | |
Function (double search_space_lower_bound, double search_space_upper_bound) | |
A constructor, specifying the lower and upper search space bounds. More... | |
Function (std::vector< double > search_space_lower_bound, std::vector< double > search_space_upper_bound) | |
A constructor specifying the lower and upper search space bounds. More... | |
virtual | ~Function () |
The destructor. More... | |
virtual mpf_t * | DistanceTo1DLocalOptimum (const std::vector< mpf_t * > &pos, int d) |
Calculates for a position and a dimension the next local optimum if only the value of the position in the specified dimension is varied and returns the distance to it. More... | |
virtual mpf_t * | DistanceTo1DLocalOptimumTernarySearch (const std::vector< mpf_t * > &pos, int d, mpf_t *start_distance) |
Calculates for a position and a dimension the next local optimum if only the value of the position in the specified dimension is varied and returns the distance to it. More... | |
virtual mpf_t * | Eval (const std::vector< mpf_t * > &pos)=0 |
Returns the evaluation of the current function at the given position. More... | |
mpf_t * | Evaluate (const std::vector< mpf_t * > &pos) |
This is the initial function for evaluation of a position. More... | |
virtual std::vector< mpf_t * > | GetLowerSearchSpaceBound () |
Returns the lower search space bound of the search space for this objective function. More... | |
virtual std::string | GetName ()=0 |
Returns a string representation of this object. More... | |
virtual std::vector< mpf_t * > | GetUpperSearchSpaceBound () |
Returns the upper search space bound of the search space for this objective function. More... | |
void | InitSearchSpaceBounds () |
Initializes the search space bounds. More... | |
void | SetFunctionBound (SearchSpaceBound *search_space_bound) |
Updates the bound of the search space according to the given data. More... | |
Detailed Description
This class is an abstract class. It supplies the interface for objective functions.
Constructor & Destructor Documentation
|
inlinevirtual |
The destructor.
highprecisionpso::Function::Function | ( | ) |
A constructor.
highprecisionpso::Function::Function | ( | double | search_space_lower_bound, |
double | search_space_upper_bound | ||
) |
A constructor, specifying the lower and upper search space bounds.
- Parameters
-
search_space_lower_bound The lower search space bound for each dimension. search_space_upper_bound The upper search space bound for each dimension.
highprecisionpso::Function::Function | ( | std::vector< double > | search_space_lower_bound, |
std::vector< double > | search_space_upper_bound | ||
) |
A constructor specifying the lower and upper search space bounds.
- Parameters
-
search_space_lower_bound The lower search space bound. search_space_upper_bound The upper search space bound.
Member Function Documentation
|
virtual |
Calculates for a position and a dimension the next local optimum if only the value of the position in the specified dimension is varied and returns the distance to it.
For a given position all values (beside the value in the specified dimension) are regarded as constants. By a ternary search, where the value of the position in the specified dimension is varied, a local optimum is calculated. The result is the distance of the evaluated position and the found local optimum.
- Parameters
-
pos The position. d The index of the observed dimension.
- Returns
- The distance to the next local optimum in a single dimensional setting.
Reimplemented in highprecisionpso::Norm1Plus2PowerMinusK, highprecisionpso::ScaledSphereRand, highprecisionpso::ScaledHadamardRotatedSphere, highprecisionpso::Norm2PowerK, highprecisionpso::InclinedPlaneAsym, highprecisionpso::NormInfinity, highprecisionpso::RandomPositiveDefiniteSecondDerivative, highprecisionpso::ScaledSphere, highprecisionpso::Schwefel1, highprecisionpso::MonoSphere, highprecisionpso::InclinedPlane, highprecisionpso::SortingFunction, highprecisionpso::TwoCupsFunction, highprecisionpso::DiagonalFunction, highprecisionpso::Norm1, and highprecisionpso::SpherePlus.
|
virtual |
Calculates for a position and a dimension the next local optimum if only the value of the position in the specified dimension is varied and returns the distance to it.
For a given position all values (beside the value in the specified dimension) are regarded as constants. By a ternary search, where the value of the position in the specified dimension is varied, a local optimum is calculated. The result is the distance of the evaluated position and the found local optimum.
- Parameters
-
pos The position. d The index of the observed dimension. start_distance The initial step length for the ternary search.
- Returns
- The distance to the next local optimum in a single dimensional setting.
|
pure virtual |
Returns the evaluation of the current function at the given position.
If you write your own function please override this function.
- Warning
- The cases where the position appears to be outside of the search space bounds is handled already in the evaluate function.
- Parameters
-
pos The position.
- Returns
- The function value of the position.
Implemented in highprecisionpso::Norm1Plus2PowerMinusK, highprecisionpso::ScaledSphereRand, highprecisionpso::ConstantFunction, highprecisionpso::CombineFunction, highprecisionpso::ScaledHadamardRotatedSphere, highprecisionpso::Norm2PowerK, highprecisionpso::SingleDifferentDirection, highprecisionpso::Schwefel2, highprecisionpso::OperatedFunction, highprecisionpso::InclinedPlaneAsym, highprecisionpso::MovedRosenbrock, highprecisionpso::NormInfinity, highprecisionpso::TestingFunction, highprecisionpso::RandomPositiveDefiniteSecondDerivative, highprecisionpso::FunctionReduceOperator, highprecisionpso::ScaledSphere, highprecisionpso::DiagonalFunction, highprecisionpso::Rastrigin, highprecisionpso::Rosenbrock, highprecisionpso::InclinedPlane, highprecisionpso::MonoSphere, highprecisionpso::TwoCupsFunction, highprecisionpso::Schwefel1, highprecisionpso::SortingFunction, highprecisionpso::Norm1, and highprecisionpso::SpherePlus.
mpf_t* highprecisionpso::Function::Evaluate | ( | const std::vector< mpf_t * > & | pos | ) |
This is the initial function for evaluation of a position.
Here the cases are handled where the position lies outside of the search space bounds.
- Warning
- Do not override this method. If you write your own function override the function eval!
- Parameters
-
pos The position which should be evaluated.
- Returns
- The function value of the position.
|
virtual |
Returns the lower search space bound of the search space for this objective function.
- Returns
- The lower bound.
|
pure virtual |
Returns a string representation of this object.
- Returns
- The name of the object.
Implemented in highprecisionpso::Norm1Plus2PowerMinusK, highprecisionpso::ScaledSphereRand, highprecisionpso::ConstantFunction, highprecisionpso::CombineFunction, highprecisionpso::ScaledHadamardRotatedSphere, highprecisionpso::Norm2PowerK, highprecisionpso::SingleDifferentDirection, highprecisionpso::Schwefel2, highprecisionpso::OperatedFunction, highprecisionpso::InclinedPlaneAsym, highprecisionpso::MovedRosenbrock, highprecisionpso::NormInfinity, highprecisionpso::TestingFunction, highprecisionpso::RandomPositiveDefiniteSecondDerivative, highprecisionpso::FunctionReduceOperator, highprecisionpso::ScaledSphere, highprecisionpso::DiagonalFunction, highprecisionpso::Rastrigin, highprecisionpso::Rosenbrock, highprecisionpso::InclinedPlane, highprecisionpso::MonoSphere, highprecisionpso::TwoCupsFunction, highprecisionpso::Schwefel1, highprecisionpso::SortingFunction, highprecisionpso::Norm1, and highprecisionpso::SpherePlus.
|
virtual |
Returns the upper search space bound of the search space for this objective function.
- Returns
- The upper bound.
void highprecisionpso::Function::InitSearchSpaceBounds | ( | ) |
Initializes the search space bounds.
void highprecisionpso::Function::SetFunctionBound | ( | SearchSpaceBound * | search_space_bound | ) |
Updates the bound of the search space according to the given data.
- Parameters
-
search_space_bound The information about the updated search space bounds.
The documentation for this class was generated from the following file:
- src/function/function.h