operations.h File Reference
This file contains a large set of operations for calculations with the mpf_t data type. More...
#include <gmp.h>
#include <string>
#include <vector>
#include "arbitrary_precision_calculation/random_number_generator.h"
Include dependency graph for operations.h:
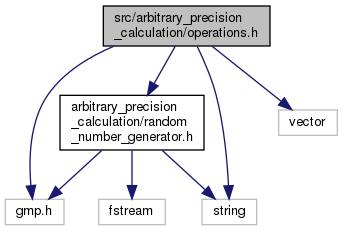
This graph shows which files directly or indirectly include this file:
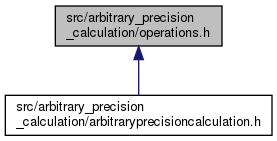
Go to the source code of this file.
Namespaces | |
arbitraryprecisioncalculation | |
base namespace for arbitrary precision calculation. | |
arbitraryprecisioncalculation::mpftoperations | |
Contains a large set of operations for calculations with the mpf_t data type. | |
arbitraryprecisioncalculation::vectoroperations | |
Contains a large set of operations for calculations with vectors of the mpf_t data type. | |
Functions | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Abs (const mpf_t *x) |
Calculates the absolute value of the supplied value. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::Add (const std::vector< mpf_t * > &a, const std::vector< mpf_t * > &b) |
Performs element wise addition of the two vectors. More... | |
mpf_t * | arbitraryprecisioncalculation::vectoroperations::Add (const std::vector< mpf_t * > &a) |
Calculates the sum of the vector entries. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Add (const mpf_t *a, const mpf_t *b) |
Performs an addition of the two values. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Arccos (mpf_t *x) |
Calculates the arccosine function of the supplied value. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Arcsin (mpf_t *x) |
Calculates the arcsine function of the supplied value. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Arctan (mpf_t *x) |
Calculates the arctangent function of the supplied value. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Ceil (mpf_t *value) |
Calculates the smallest integral value greater or equal than the supplied value. More... | |
void | arbitraryprecisioncalculation::mpftoperations::ChangeNumberOfMpftValuesCached (int change) |
Changes the number of mpf_t values currently in cache by the specified number. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::Clone (const std::vector< mpf_t * > &a) |
Creates a copy of the supplied vector where each entry is cloned. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Clone (const mpf_t *value) |
Creates a copy of the supplied value. More... | |
int | arbitraryprecisioncalculation::mpftoperations::Compare (const mpf_t *a, const mpf_t *b) |
Compares the two parameters. More... | |
int | arbitraryprecisioncalculation::mpftoperations::Compare (const double a, const mpf_t *b) |
Compares the two parameters. More... | |
int | arbitraryprecisioncalculation::mpftoperations::Compare (const mpf_t *a, double b) |
Compares the two parameters. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Cos (mpf_t *x) |
Calculates the cosine function of the supplied value. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::Divide (const std::vector< mpf_t * > &a, const std::vector< mpf_t * > &b) |
Performs element wise division of the two vectors. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Divide (const mpf_t *a, const mpf_t *b) |
Performs a division of the two values. More... | |
bool | arbitraryprecisioncalculation::vectoroperations::Equals (const std::vector< mpf_t * > &a, const std::vector< mpf_t * > &b) |
Checks whether two mpf_t* vectors are equal. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Exp (mpf_t *a) |
Calculates the exponential function of the input. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Floor (mpf_t *value) |
Calculates the largest integral value less or equal than the supplied value. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::GetConstantVector (int dimension, const double &value) |
Creates a vector with the specified number of entries. Each entry represents the supplied value. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::GetConstantVector (int dimension, const mpf_t *value) |
Creates a vector with the specified number of entries. Each entry represents the supplied value. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetE () |
Calculates the value of E. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetGaussianRandomMpft (double mu, double sigma, RandomNumberGenerator *random) |
Calculates a random value with normal distribution (gaussian distribution). More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetGaussianRandomMpft (double mu, double sigma) |
Calculates a random value with normal distribution (gaussian distribution). More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetMinusInfinity () |
Calculates the representation of -infinity. More... | |
int | arbitraryprecisioncalculation::mpftoperations::GetNumberOfMpftValuesCached () |
Getter function for the number of mpf_t values which are currently cached. More... | |
int | arbitraryprecisioncalculation::mpftoperations::GetNumberOfMpftValuesInUse () |
Getter function for the number of mpf_t values which are currently in use. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetPi () |
Calculates the value of Pi. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetPlusInfinity () |
Calculates the representation of +infinity. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetRandomMpft (RandomNumberGenerator *random) |
Calculates a random mpf_t value in the range [0,1] with the supplied random number generator. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetRandomMpft () |
Calculates a random mpf_t value in the range [0,1] with the specified standard random number generator for this execution. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::GetRandomVector (int dimensions) |
Creates a random mpf_t* vector with the specified number of entries, which contains random values in the interval [0,1]. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetResultPointer () |
Prepares an mpf_t value for using it. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::GetUndefined () |
Calculates the representation of an undefined value. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::GetVector (const std::vector< double > &a) |
Transforms the vector with double entries to a vector with mpf_t* values. More... | |
void | arbitraryprecisioncalculation::mpftoperations::IncreasePrecision () |
Increases the precision instantly to a higher value. More... | |
bool | arbitraryprecisioncalculation::mpftoperations::IsInfinite (const mpf_t *value) |
Checks whether the supplied value is the representation of +infinity or -infinity. More... | |
bool | arbitraryprecisioncalculation::mpftoperations::IsMinusInfinity (const mpf_t *value) |
Checks whether the supplied value is the representation of -infinity. More... | |
bool | arbitraryprecisioncalculation::mpftoperations::IsPlusInfinity (const mpf_t *value) |
Checks whether the supplied value is the representation of +infinity. More... | |
bool | arbitraryprecisioncalculation::mpftoperations::IsUndefined (const mpf_t *value) |
Checks whether the supplied value is the representation of an undefined value. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::LoadMpft (std::ifstream *stream) |
Recovers a mpf_t value from an input stream. More... | |
double | arbitraryprecisioncalculation::mpftoperations::Log2Double (mpf_t *value) |
Calculates the base 2 logarithm of the specified value. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::LogE (mpf_t *value) |
Calculates the base E logarithm of the specified value. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Max (mpf_t *a, mpf_t *b) |
Calculates the maximum of the two values. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Min (mpf_t *a, mpf_t *b) |
Calculates the minimum of the two values. More... | |
std::vector< double > | arbitraryprecisioncalculation::vectoroperations::MpftToDouble (const std::vector< mpf_t * > &a) |
Converts the values of a vector with mpf_t* entries to a vector with double entries. More... | |
double | arbitraryprecisioncalculation::mpftoperations::MpftToDouble (mpf_t *value) |
Converts an mpf_t value to a double value. More... | |
std::string | arbitraryprecisioncalculation::mpftoperations::MpftToString (const mpf_t *value) |
Converts an mpf_t value to a string representation of it. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::Multiply (const std::vector< mpf_t * > &a, const std::vector< mpf_t * > &b) |
Performs element wise multiplication of the two vectors. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::Multiply (const std::vector< mpf_t * > &a, const std::vector< double > &b) |
Performs element wise multiplication of the two vectors. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::Multiply (const std::vector< mpf_t * > &a, const double &b) |
Performs for each element in the input vector a multiplication with the specified factor. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::Multiply (const std::vector< mpf_t * > &a, mpf_t *b) |
Performs for each element in the input vector a multiplication with the specified factor. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Multiply (const mpf_t *a, const mpf_t *b) |
Performs a multiplication of the two values. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Multiply (const mpf_t *a, const double b) |
Performs a multiplication of the two values. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Multiply2Exp (const mpf_t *a, int p) |
Multiplies the value of parameter a by a factor of 2p. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Negate (const mpf_t *x) |
Calculates the negation of the supplied value. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::OrthogonalProjection (const std::vector< mpf_t * > &vec, const std::vector< mpf_t * > &projection_vector) |
Calculates the orthogonal projection of the vector vec on the projection vector. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Pow (mpf_t *value, int exponent) |
Calculates the value to the power of the specified exponent. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Pow (mpf_t *value, double exponent) |
Calculates the value to the power of the specified exponent. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Pow (mpf_t *value, mpf_t *exponent) |
Calculates the value to the power of the specified exponent. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::Randomize (const std::vector< mpf_t * > &a) |
Randomizes the supplied vector. Each entry will be multiplied by a random mpf_t value in the interval [0,1]. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Randomize (mpf_t *value) |
Multiplies the supplied value by a random value in the interval [0,1]. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Randomize (mpf_t *value, RandomNumberGenerator *random) |
Multiplies the supplied value by a random value in the interval [0,1]. More... | |
void | arbitraryprecisioncalculation::mpftoperations::ReleaseValue (mpf_t *a) |
Releases the reserved memory for the supplied mpf_t. More... | |
void | arbitraryprecisioncalculation::vectoroperations::ReleaseValues (const std::vector< mpf_t * > &a) |
Release the reserved memory for the entries of the supplied vector. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Sin (mpf_t *x) |
Calculates the sine function of the supplied value. More... | |
void | arbitraryprecisioncalculation::vectoroperations::Sort (std::vector< mpf_t * > *vec) |
Sorts the elements of the supplied vector in increasing order. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Sqrt (mpf_t *value) |
Calculates the square root of the specified value. More... | |
mpf_t * | arbitraryprecisioncalculation::vectoroperations::SquaredEuclideanLength (const std::vector< mpf_t * > &vec) |
Calculates the squared euclidean length of the supplied vector. More... | |
void | arbitraryprecisioncalculation::mpftoperations::StatisticalCalculationsEnd () |
Signals the end of statistical calculations. More... | |
void | arbitraryprecisioncalculation::mpftoperations::StatisticalCalculationsStart () |
Signals the start of statistical calculations. More... | |
void | arbitraryprecisioncalculation::mpftoperations::StoreMpft (mpf_t *value, std::ofstream *stream) |
Writes the content of a mpf_t value to the given stream such that it can completely recovered. More... | |
std::vector< mpf_t * > | arbitraryprecisioncalculation::vectoroperations::Subtract (const std::vector< mpf_t * > &a, const std::vector< mpf_t * > &b) |
Performs element wise subtraction of the two vectors. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Subtract (const mpf_t *a, const mpf_t *b) |
Performs a subtraction of the two values. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::Tan (mpf_t *x) |
Calculates the tangent function of the supplied value. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::ToMpft (double value) |
Converts the supplied double value to its mpf_t representation. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::ToMpft (long long value) |
Converts the supplied long long value to its mpf_t representation. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::ToMpft (unsigned long long value) |
Converts the supplied unsigned long long value to its mpf_t representation. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::ToMpft (int value) |
Converts the supplied int value to its mpf_t representation. More... | |
mpf_t * | arbitraryprecisioncalculation::mpftoperations::ToMpft (unsigned int value) |
Converts the supplied unsigned int value to its mpf_t representation. More... | |
Detailed Description
This file contains a large set of operations for calculations with the mpf_t data type.
- Date
- July, 2013
- Copyright
- This project is released under the MIT License (MIT).
- The MIT License (MIT)
- Copyright (c) 2016 by Friedrich-Alexander-Universität Erlangen-Nürnberg and Alexander Raß
- Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
- The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
- THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.