configuration.h File Reference
This file contains general information about the configuration for running the particle swarm optimization algorithm. More...
#include <string>
#include <vector>
#include "bound_handling/bound_handling.h"
#include "function/function.h"
#include "neighborhood/neighborhood.h"
#include "position_and_velocity_updater/position_and_velocity_updater.h"
#include "arbitrary_precision_calculation/random_number_generator.h"
#include "statistics/direct_statistics.h"
#include "statistics/statistics.h"
#include "velocity_adjustment/velocity_adjustment.h"
Include dependency graph for configuration.h:
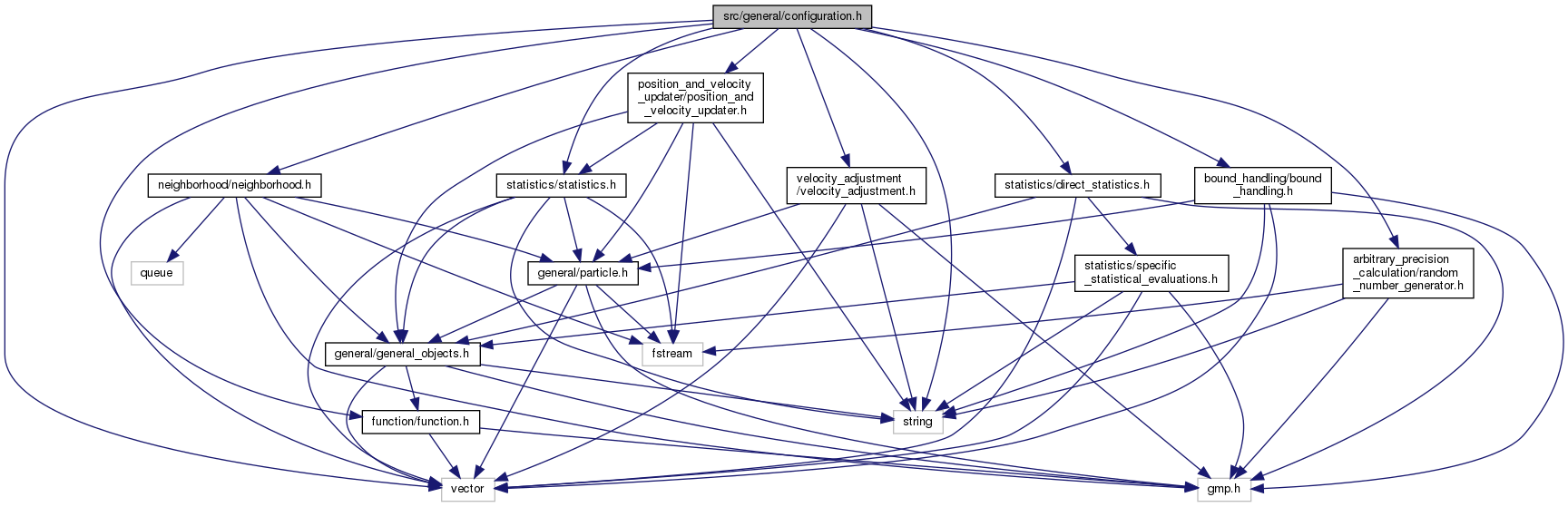
Go to the source code of this file.
Classes | |
class | highprecisionpso::configuration::InitializationInformation |
Stores the information how positions or velocities should be initialized. More... | |
Namespaces | |
highprecisionpso | |
base namespace for this project. | |
highprecisionpso::configuration | |
Contains the variables which store information about the configuration for the particle swarm optimization algorithm (PSO). | |
Functions | |
std::string | highprecisionpso::configuration::GetConfigurationString () |
Generates a short string, which includes the main information about the current configuration. More... | |
std::string | highprecisionpso::configuration::GetFilePrefix () |
If a file prefix is already specified then this file prefix is returned. Otherwise the file prefix is generated. More... | |
void | highprecisionpso::configuration::Init () |
Resets all variables in the configuration section to the default values. More... | |
bool | highprecisionpso::configuration::ReadConfigurationFile (std::string fileName) |
Reads data from the given configuration file and stores them in the configuration section. More... | |
Variables | |
BoundHandling * | highprecisionpso::configuration::g_bound_handling |
Specifies the bound handling method. More... | |
double | highprecisionpso::configuration::g_chi |
Inertia weight of PSO for movement equation. More... | |
double | highprecisionpso::configuration::g_coefficient_global_attractor |
Coefficient for the weight of the direction to the global attractor for the movement equation. More... | |
double | highprecisionpso::configuration::g_coefficient_local_attractor |
Coefficient for the weight of the direction to the local attractor for the movement equation. More... | |
bool | highprecisionpso::configuration::g_debug_swarm_activated |
Specifies whether the debug swarm mode is activated. More... | |
unsigned int | highprecisionpso::configuration::g_debug_swarm_global_attractor_trajectory |
Specifies how many of the previous global attractor positions are visualized. More... | |
std::string | highprecisionpso::configuration::g_debug_swarm_gnuplot_terminal |
Specifies the gnuplot terminal for image generation. More... | |
std::string | highprecisionpso::configuration::g_debug_swarm_image_file_extension |
Specifies the file extension for image generation with gnuplot. More... | |
unsigned int | highprecisionpso::configuration::g_debug_swarm_image_generation_frequency |
Specifies how often images are created. More... | |
bool | highprecisionpso::configuration::g_debug_swarm_show_global_attractor_on_terminal |
Specifies whether the position value and the function value of the particle swarm should be displayed at each iteration. More... | |
unsigned int | highprecisionpso::configuration::g_debug_swarm_x_resolution |
Specifies width in pixels of generated images. More... | |
unsigned int | highprecisionpso::configuration::g_debug_swarm_y_resolution |
Specifies height in pixels of generated images. More... | |
int | highprecisionpso::configuration::g_dimensions |
The number of dimensions of the search space for the particle swarm optimization algorithm. More... | |
std::string | highprecisionpso::configuration::g_file_prefix |
File prefix for all generated files. (Will be initialized in the first call of getFilePrefix) More... | |
Function * | highprecisionpso::configuration::g_function |
Specifies the objective function. More... | |
FunctionBehaviorOutsideOfBoundsMode | highprecisionpso::configuration::g_function_behavior_outside_of_bounds_mode |
Stores the information how the objective function is evaluated outside of the search space bounds. More... | |
std::vector< SearchSpaceBound > | highprecisionpso::configuration::g_function_bounds |
A list of search space bounds for the current objective function. More... | |
InitializeVelocityMode | highprecisionpso::configuration::g_initialize_velocity_mode |
Stores the information how the velocity should be initialized. More... | |
std::vector< std::vector< long long > > | highprecisionpso::configuration::g_iterations_with_statistic_evaluations |
Specifies at which steps / iterations statistical evaluations are done. Each vector entry contains 3 long long values which specify start iteration, end iteration and periodicity. More... | |
long long | highprecisionpso::configuration::g_max_iterations |
The number of iterations / steps for the particle swarm optimization algorithm. Specifies how many iterations of the particle swarm are done. More... | |
Neighborhood * | highprecisionpso::configuration::g_neighborhood |
Specifies the neighborhood topology. More... | |
int | highprecisionpso::configuration::g_particles |
The number of particles for the particle swarm optimization algorithm. More... | |
PositionAndVelocityUpdater * | highprecisionpso::configuration::g_position_and_velocity_updater |
Specifies the way how the positions and the velocities are updated at each iteration. More... | |
std::vector< InitializationInformation > | highprecisionpso::configuration::g_position_initialization_informations |
Initialization informations for position initialization. More... | |
std::vector< long long > | highprecisionpso::configuration::g_preserve_backup_times |
Contains a list of time steps at which backups of the current situation (positions, velocities, ... ) are stored. More... | |
std::string | highprecisionpso::configuration::g_run_check_configuration_file |
File name of the run check configuration file. If this value is the empty string then no run check is done. More... | |
bool | highprecisionpso::configuration::g_show_system_time_in_file_prefix |
Specifies whether the time and the date should be included in the generated files. More... | |
Statistics * | highprecisionpso::configuration::g_statistics |
Stores information about the current swarm situation. More... | |
std::vector< Statistic * > | highprecisionpso::configuration::g_statistics_list |
Contains a list of statistics which should be evaluated. More... | |
double | highprecisionpso::configuration::g_time_between_backups |
Specifies periodicity in seconds of the creation of backups and the continuation of statistics. More... | |
double | highprecisionpso::configuration::g_time_between_run_checks |
Specifies periodicity in seconds of checking whether the program is allowed to run or not. (see run check configuration in configuration files) More... | |
UpdateGlobalAttractorMode | highprecisionpso::configuration::g_update_global_attractor_mode |
Stores the information how often the global attractor is updated. More... | |
std::string | highprecisionpso::configuration::g_user_defined_file_prefix |
User defined part of the file prefix. It is empty if the user has not specified it. More... | |
VelocityAdjustment * | highprecisionpso::configuration::g_velocity_adjustment |
Specifies the method for velocity adjustment in case that the bound handling method was active. More... | |
std::vector< InitializationInformation > | highprecisionpso::configuration::g_velocity_initialization_informations |
Initialization informations for velocity initialization. More... | |
Detailed Description
This file contains general information about the configuration for running the particle swarm optimization algorithm.
- Date
- June, 2013
- Copyright
- This project is released under the MIT License (MIT).
- The MIT License (MIT)
- Copyright (c) 2016 by Friedrich-Alexander-Universität Erlangen-Nürnberg and Alexander Raß
- Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
- The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
- THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.